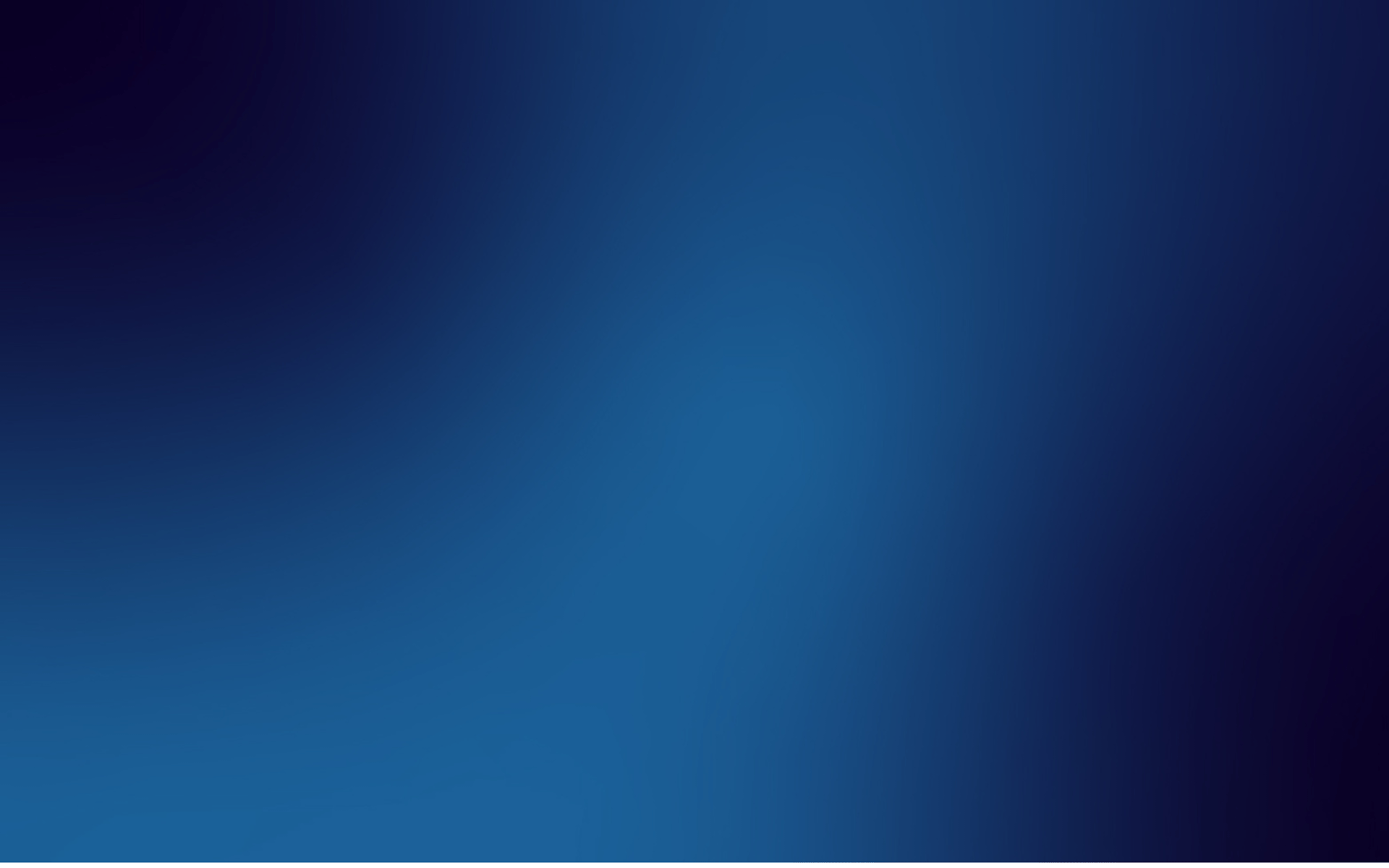
JavaScript Beyond the Basics Advanced Concepts and Techniques
By AZ Konnect Team
6/6/20242 min read
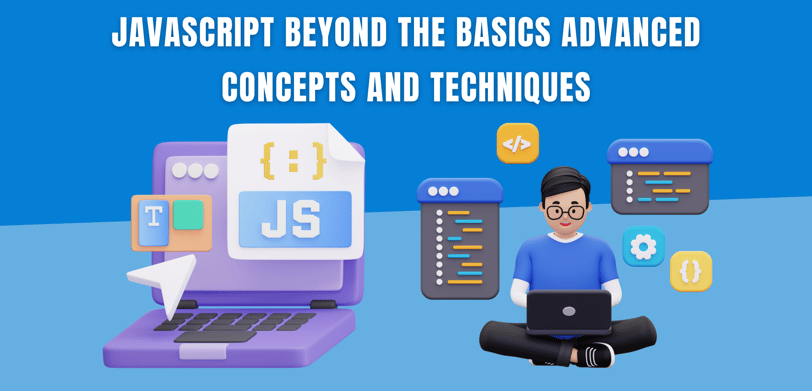
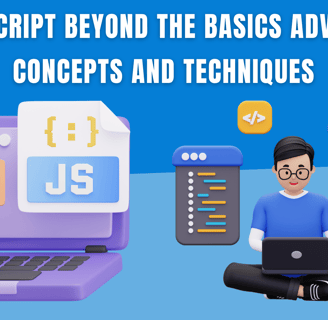
JavaScript is an essential tool for modern web development, powering everything from interactive user interfaces to server-side applications. While mastering the basics is crucial, advancing your JavaScript skills can open up new possibilities and efficiencies in your development workflow. In this blog post, we'll explore some advanced JavaScript concepts and techniques that will take your coding skills to the next level.
1. Closures
Understanding Closures: Closures are functions that have access to variables from another function’s scope. This powerful feature allows for the creation of private variables and encapsulation. Closures are commonly used in scenarios where you need to maintain state across multiple function calls or to create function factories.
2. Promises and Asynchronous Programming
Promises: Promises are used to handle asynchronous operations. They represent the eventual completion (or failure) of an asynchronous operation and its resulting value. Using promises helps to avoid callback hell and makes code more readable and maintainable.
Async/Await: Async/await syntax simplifies working with promises. It allows you to write asynchronous code that looks and behaves like synchronous code, which makes it easier to read and debug. Async/await is particularly useful for handling complex asynchronous flows where multiple promises need to be chained together.
3. Higher-Order Functions
Definition: Higher-order functions are functions that take other functions as arguments or return functions as their result. They are a key aspect of functional programming in JavaScript. Higher-order functions enable more abstract and concise code, allowing for powerful patterns like function composition and partial application.
4. Currying
Concept: Currying is the process of transforming a function with multiple arguments into a sequence of functions, each with a single argument. This technique enables the creation of more modular and reusable code. Currying is useful in scenarios where you need to fix some arguments of a function and generate a new function with fewer parameters.
5. Prototypes and Inheritance
Prototype Chain: Understanding the prototype chain is essential for mastering JavaScript’s inheritance model. Every object in JavaScript has a prototype, which is another object from which it inherits properties and methods. Prototypes allow for shared behavior and efficient memory usage. Mastering prototypes and inheritance is crucial for creating complex objects and using JavaScript's object-oriented features effectively.
6. Modules
ES6 Modules: Modules allow you to break your code into reusable pieces. With the introduction of ES6, JavaScript now has a standardized module system, which helps in organizing and maintaining large codebases. Modules improve code reusability, readability, and maintainability by encapsulating functionality and exposing only what is necessary.
Exporting and Importing: You can export variables, functions, or classes from one module and import them into another. This modular approach allows developers to create libraries of reusable code and manage dependencies more effectively.
Conclusion
Advancing your JavaScript skills beyond the basics involves mastering closures, promises, higher-order functions, currying, prototypes, and modules. These concepts and techniques not only enhance your coding proficiency but also enable you to write more efficient, maintainable, and scalable code. Embrace these advanced JavaScript features to unlock new possibilities and elevate your development capabilities.
Subscribe To Our Newsletter
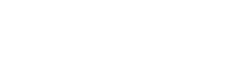
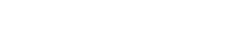