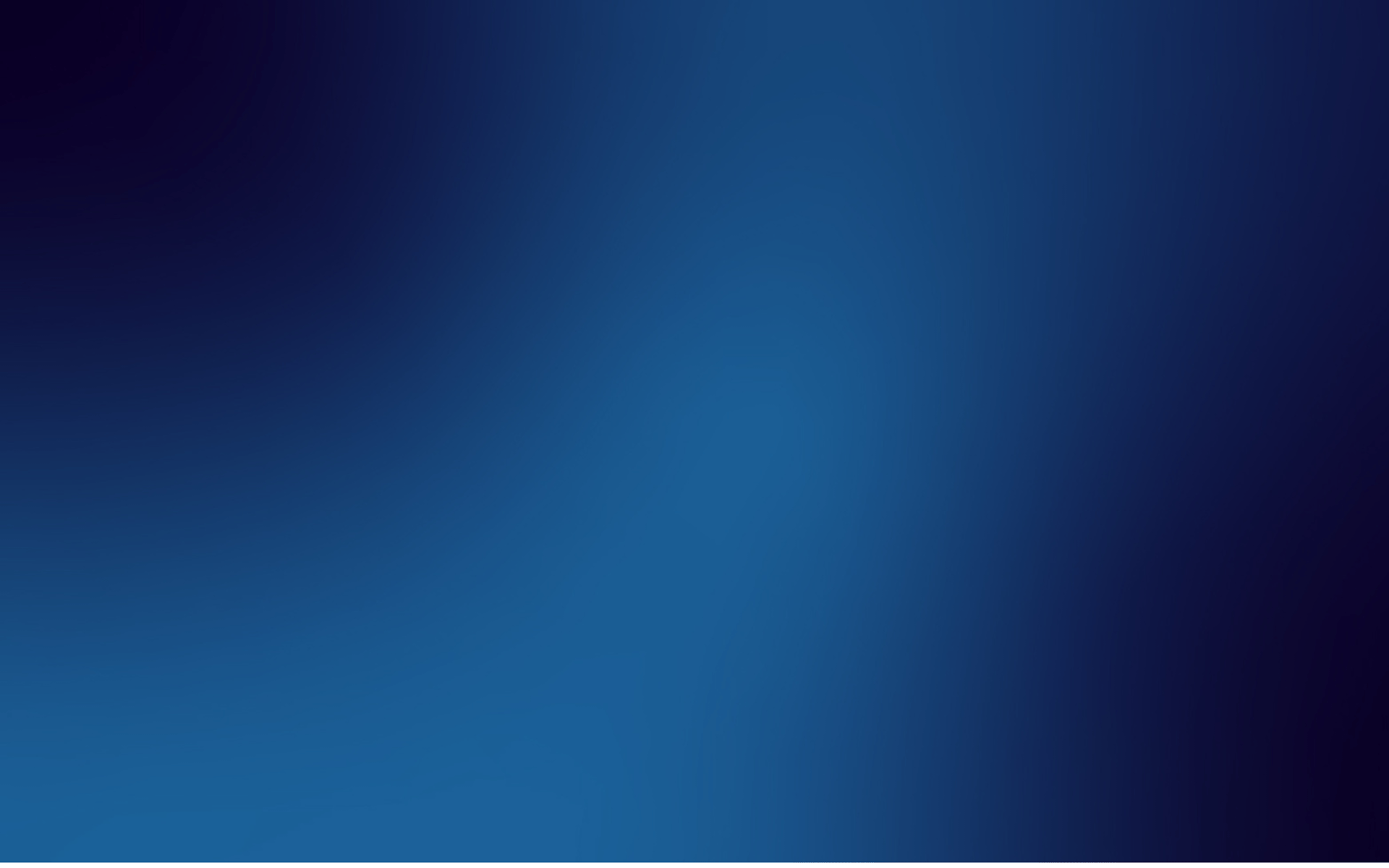
Web Components Reusable UI Elements for Modern Development
By AZ Konnect Team
12/10/20246 min read
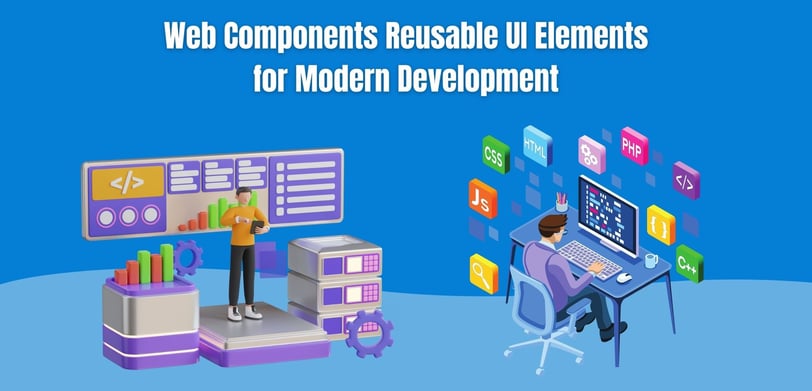
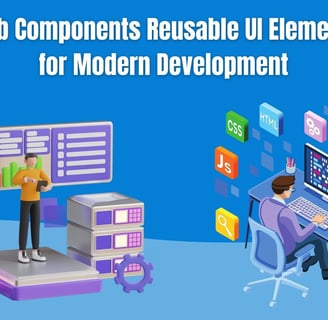
In the rapidly evolving landscape of web development, efficiency, scalability, and maintainability are key to building successful web applications. One approach that has gained significant traction in recent years is the use of Web Components, a set of standardized technologies that allow developers to create reusable UI elements that work seamlessly across different projects and frameworks.
Web Components provide a powerful solution to many of the challenges faced by modern developers, including maintaining consistency, reducing code duplication, and enabling cross-platform compatibility. In this blog post, we’ll explore what Web Components are, how they work, and how they can benefit developers by streamlining the process of building modular, reusable elements for today’s web applications.
1. What Are Web Components?
Web Components are a set of web standards that allow developers to create self-contained, reusable elements using custom HTML tags. These components encapsulate their own structure (HTML), style (CSS), and behavior (JavaScript), making them independent of the rest of the application’s code. This modular approach enables developers to build user interface (UI) elements that can be easily reused across multiple pages or even different projects without worrying about conflicts or dependencies.
The Three Core Technologies of Web Components:
Custom Elements:
This allows developers to define their own HTML tags (e.g., `<my-button>`, `<user-profile>`) and associate them with a specific behavior or functionality. Custom elements are the foundation of Web Components, enabling developers to extend the capabilities of HTML.
Shadow DOM:
The Shadow DOM provides encapsulation for a Web Component’s internal structure and style. This means that the styles and DOM elements within the component are isolated from the rest of the page, preventing style clashes or unintended behavior. The Shadow DOM ensures that a component looks and behaves the same, regardless of where it’s used.
HTML Templates:
HTML templates are reusable pieces of HTML markup that can be defined and later instantiated by JavaScript. These templates are not rendered until they are explicitly called, allowing developers to create dynamic content that can be inserted into the DOM on demand. Combined with Custom Elements, templates enable the creation of rich, dynamic UI components.
2. Why Use Web Components?
Web Components offer a variety of benefits that make them an attractive option for developers looking to build scalable, maintainable web applications. From reducing code duplication to ensuring consistency across projects, here are some key reasons why Web Components have become an essential tool for modern development:
1. Reusability
One of the main advantages of Web Components is their reusability. Since Web Components are self-contained and independent of the rest of the codebase, they can be easily reused across different parts of an application or even in entirely different projects. This means you can build a component once and use it multiple times without worrying about conflicts or rewriting the same code.
2. Encapsulation
The Shadow DOM provides true encapsulation for Web Components, ensuring that the internal structure and style of a component are completely isolated from the global page styles. This prevents issues like CSS leaking into other parts of the application or unintended DOM manipulation. With encapsulation, developers can confidently use their components without fear of breaking other parts of the app.
3. Framework Agnostic
Unlike many popular UI libraries and frameworks (such as React, Angular, or Vue), Web Components are framework-agnostic. This means that they work natively in any modern browser and can be used alongside any JavaScript framework—or none at all. This flexibility makes Web Components an ideal choice for teams working with different technologies or looking to future-proof their applications.
4. Modularity
Web Components promote modularity in web development, making it easier to build and maintain complex UIs. Each component can be developed, tested, and updated independently of the rest of the application. This modular approach reduces the complexity of the codebase, making it easier to manage and scale as the project grows.
5. Consistency
By defining UI elements as reusable components, developers can ensure a consistent look and feel across their entire application. This is particularly useful for design systems, where components like buttons, forms, and navigation elements need to maintain uniformity across different parts of the app. Web Components help developers enforce consistency by centralizing the development and maintenance of these common elements.
3. How to Create a Web Component
Creating a Web Component involves defining a custom element, encapsulating it with the Shadow DOM, and optionally using HTML templates for its structure. Below is a basic example of how to create a simple button component using Web Components.
Example:
Creating a Custom Button Component
```javascript
// Define a class for the new custom element
class MyButton extends HTMLElement {
constructor() {
super(); // Always call super first in the constructor.
// Attach a shadow DOM to the component
const shadow = this.attachShadow({ mode: 'open' });
// Create a button element
const button = document.createElement('button');
button.textContent = 'Click Me!';
// Create some styles for the button
const style = document.createElement('style');
style.textContent = `
button {
background-color: #4CAF50;
color: white;
padding: 10px 20px;
border: none;
cursor: pointer;
}
button:hover {
background-color: #45a049;
}
`;
// Append the button and style to the shadow DOM
shadow.appendChild(style);
shadow.appendChild(button);
}}
// Define the new custom element
customElements.define('my-button', MyButton);
```
In this example, we define a custom element called `<my-button>` using JavaScript’s `class` syntax. The `attachShadow` method creates a shadow DOM, which is where the component’s internal structure and styles are applied. The component itself consists of a simple button with styles that are isolated from the rest of the page.
Once defined, you can use this component in your HTML like any other native HTML element:
```html
<my-button></my-button>
```
This will render a button with the styles and functionality encapsulated within the component.
4. Real-World Use Cases for Web Components
Web Components are particularly useful in scenarios where consistency, reusability, and modularity are critical. Here are a few real-world use cases where Web Components can shine:
1. Design Systems
Design systems often include a library of reusable UI components such as buttons, modals, and form inputs. Web Components allow these elements to be created once and reused across multiple projects or teams, ensuring a consistent user experience while reducing development time.
2. Micro Frontends
In the world of microservices and micro frontends, different parts of an application may be built by different teams using different technologies. Web Components provide a way to create UI elements that can be shared across these different frontends, regardless of the underlying framework, making them a perfect fit for this architecture.
3. Cross-Platform Applications
For applications that need to run on different platforms (e.g., web, mobile, desktop), Web Components can help ensure that UI elements are consistent across all environments. By building reusable components that work across various platforms, developers can reduce duplication and streamline updates.
4. E-commerce Platforms
E-commerce sites often use reusable elements such as product cards, reviews, and checkout forms. By leveraging Web Components, e-commerce platforms can create reusable and easily maintainable elements that can be updated in one place and reflected across the entire site.
5. Challenges and Considerations
While Web Components offer many benefits, there are a few challenges and considerations that developers should be aware of:
Browser Support:
Modern browsers like Chrome, Firefox, and Edge fully support Web Components, but older browsers (such as Internet Explorer) may require polyfills. Developers need to assess the browser requirements of their user base before adopting Web Components.
Learning Curve:
For developers who are new to the concept of encapsulation, Shadow DOM, and Custom Elements, there may be a learning curve. However, the advantages of reusability and modularity often outweigh the initial investment in learning these technologies.
Performance Considerations:
While Web Components themselves are lightweight, using a large number of components with complex shadow DOM structures can impact performance, particularly on low-powered devices. Performance optimization should be considered when working with many Web Components in a single application.
Conclusion
Web Components represent a modern, efficient approach to building reusable UI elements for web applications. By encapsulating HTML, CSS, and JavaScript in modular components, developers can create scalable, maintainable, and framework-agnostic elements that work seamlessly across different projects.
Whether you’re building a design system, a micro frontend architecture, or simply looking to enhance the reusability of your UI components, Web Components provide a powerful toolset for modern development. As browser support continues to grow and the web development ecosystem evolves, Web Components are set to play an increasingly important role in shaping the future of web development.
By embracing Web Components, developers can not only save time but also ensure their applications are more modular, maintainable, and ready to meet the demands of the modern web.
Subscribe To Our Newsletter
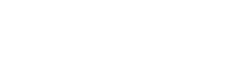
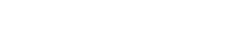